It might feel a little weird but NFTs can be repurposed as soulbound tokens. This means that the ERC-721 and ERC-1155 can be repurposed as soulbound token standard.
Logic Behind Using ERC-721 and ERC-1155 as Soulbound Token Standard
Soulbound Tokens are more or less, non-transferable NFTs that are attached to a specific wallet at minting. They only serve the functionality of identification in Web3. Therefore, developing a separate token standard might seem a little far fetched such application.
ERC-721 is the Non-Fungible token standard which supports the creation of one token per smart contract. ERC-1155 is the Semi-Fungible token standard which supports the creation of batch of tokens per smart contract. Now, whether to use ERC-721 or the ERC-1155 is on the developer. ERC-721 can be easily created using tools and without any coding experience while ERC-1155 required more coding knowledge. There are other differentiating features as well.
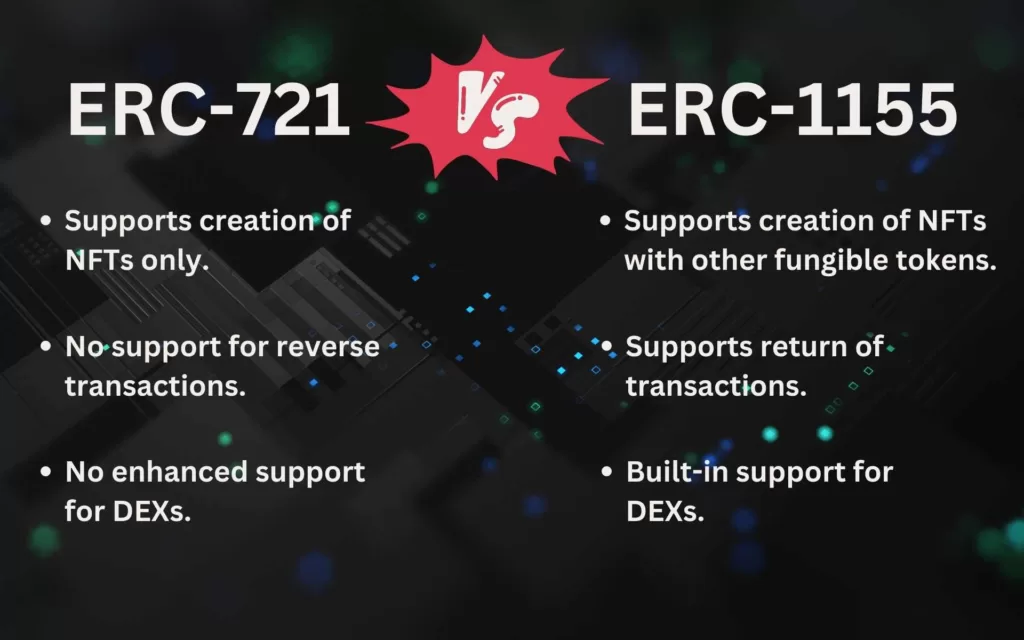
How Can I Implement ERC-721 or ERC-1155 as a Soulbound Token Standard
Below is a code generated by an AI Tool which features an NFT smart contract but without any token transfer ability. You can use this code to understand the logic of using NFTs as soulbound token standard.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract NonTransferableNFT {
address private owner;
string private tokenURI;
event OwnershipTransferred(address indexed previousOwner, address indexed newOwner);
constructor(string memory _tokenURI) {
owner = msg.sender;
tokenURI = _tokenURI;
}
modifier onlyOwner() {
require(msg.sender == owner, "Only the owner can perform this action.");
_;
}
function transferOwnership(address _newOwner) public onlyOwner {
require(_newOwner != address(0), "Invalid address.");
emit OwnershipTransferred(owner, _newOwner);
owner = _newOwner;
}
function getTokenURI() public view returns (string memory) {
return tokenURI;
}
function setTokenURI(string memory _newTokenURI) public onlyOwner {
tokenURI = _newTokenURI;
}
}
Specific Soulbound Token Standards
There are two token standards that are currently focused only on Soulbound Tokens, the ERC-5114 and ERC 5484. These two token standards are currently under review and can change depending upon further inputs by the Ethereum community.
ERC-5114
It is a soulbound badge token standard. It was first proposed by Micah Zoltu on May 30, 2022. As of today, on July 10, 2023, it is under final review of the Ethereum community.
Sample Code from Ethereum.org for ERC-5114 Soulbound Token Standard
// SPDX-License-Identifier: CC0-1.0
pragma solidity ^0.8.0;
interface IERC5484 {
/// A guideline to standardlize burn-authorization's number coding
enum BurnAuth {
IssuerOnly,
OwnerOnly,
Both,
Neither
}
/// @notice Emitted when a soulbound token is issued.
/// @dev This emit is an add-on to nft's transfer emit in order to distinguish sbt
/// from vanilla nft while providing backward compatibility.
/// @param from The issuer
/// @param to The receiver
/// @param tokenId The id of the issued token
event Issued (
address indexed from,
address indexed to,
uint256 indexed tokenId,
BurnAuth burnAuth
);
/// @notice provides burn authorization of the token id.
/// @dev unassigned tokenIds are invalid, and queries do throw
/// @param tokenId The identifier for a token.
function burnAuth(uint256 tokenId) external view returns (BurnAuth);
}
ERC-5484
It is the token standard for Consensual Soulbound Token. It was designed by Buzz Cai on August 17, 2022. As of today on July 10, 2023, the token standard is finalized and live on the Ethereum community.
Sample Code from Ethereum.org for ERC-5484 Soulbound Token Standard
interface IERC5114 {
// fired anytime a new instance of this badge is minted
// this event **MUST NOT** be fired twice for the same `badgeId`
event Mint(uint256 indexed badgeId, address indexed nftAddress, uint256 indexed nftTokenId);
// returns the NFT that this badge is bound to.
// this function **MUST** throw if the badge hasn't been minted yet
// this function **MUST** always return the same result every time it is called after it has been minted
// this function **MUST** return the same value as found in the original `Mint` event for the badge
function ownerOf(uint256 badgeId) external view returns (address nftAddress, uint256 nftTokenId);
// returns a URI with details about this badge collection
// the metadata returned by this is merged with the metadata return by `badgeUri(uint256)`
// the collectionUri **MUST** be immutable (e.g., ipfs:// and not http://)
// the collectionUri **MUST** be content addressable (e.g., ipfs:// and not http://)
// data from `badgeUri` takes precedence over data returned by this method
// any external links referenced by the content at `collectionUri` also **MUST** follow all of the above rules
function collectionUri() external pure returns (string collectionUri);
// returns a censorship resistant URI with details about this badge instance
// the collectionUri **MUST** be immutable (e.g., ipfs:// and not http://)
// the collectionUri **MUST** be content addressable (e.g., ipfs:// and not http://)
// data from this takes precedence over data returned by `collectionUri`
// any external links referenced by the content at `badgeUri` also **MUST** follow all of the above rules
function badgeUri(uint256 badgeId) external view returns (string badgeUri);
// returns a string that indicates the format of the `badgeUri` and `collectionUri` results (e.g., 'EIP-ABCD' or 'soulbound-schema-version-4')
function metadataFormat() external pure returns (string format);
}